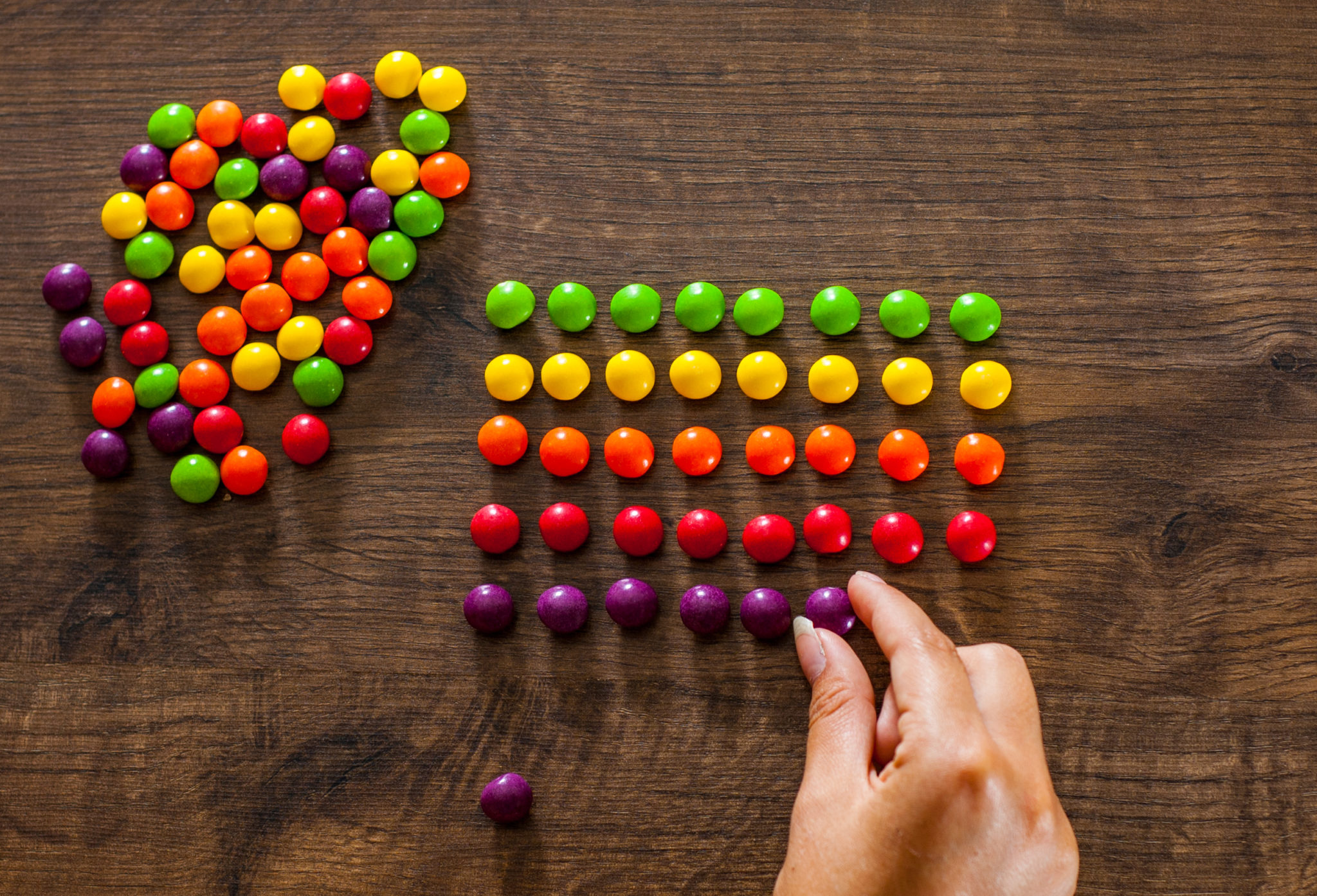
Sorting Visualization
I build a sorting visualization tool: Check it out What Technologies I used Github Pages (Hosting & Deployment) D3.js (Visualization) Javascript (UI & Sorting Logic) HTML (UI) CSS (Styling) How did I build It Code All of the code available on Github. Data Object The SortData type serves as a wrapper that contains all the necessary information for visualizing the sorting algorithm. type SortData = { init_index: number, value: number, color: string } Data Validation and Object Creation Helper functions The validateData function validates the user input data to ensure that it is in the correct format and can be processed....